2025-06-13 | Maven 3.9.10 |
2025-04-28 | TeX-UPmethodology 20250428 |
2025-04-26 | TeX-UPmethodology 20250426 |
2024-09-24 | Maven 3.9.9 |
2024-08-13 | Noble Numbat |
2024-06-21 | Maven 3.9.8 |
This page contains a tutorial that permits to create a simple Finite State Machine based on the NetEditor library.
The definition of a new language's editor should follow the steps:
- definition of the language constructs
- definition of the figures
- definition of the factories
- graphical user interface
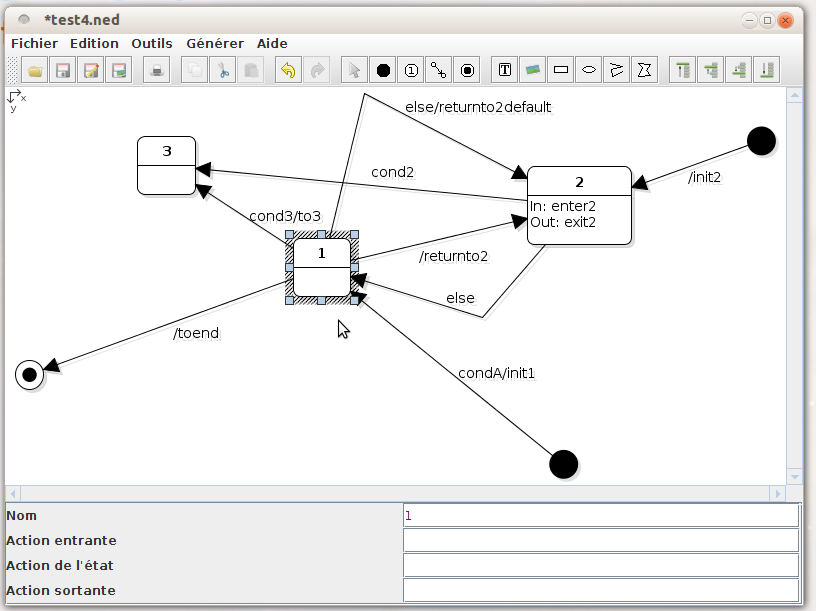
1. Definition of the language constructs
The maven module related to this step is: org.arakhne.neteditor.fsm:fsm-constructs
.
The Finite State Machine language is composed of the constructs: state, transitions, start point, end point.
1.1. Definition of the abstraction for all the nodes of the FSM
The FSM diagram contains three types of nodes: state, end point, and start point. It is recommended to create an abstract class that is common to all the implementations of these nodes. Each node of an FSM contains only one anchor.
The AbstractFSMNode
class extends the class StandardMonoAnchorNode
, which is the implementation of a node with a single anchor inside. The generic parameters are the types of the graph, node, anchor and transition supported by the implementation (here the FSM classes). The constructor of the super class takes an instance of anchor as parameter. The FSMAnchor is used.
The AbstractFSMNode
class permits to simplify the use of the generic parameters.
1.2. State Definition
The FSM state is defined in the class FSMState
. A state has a name, an action to execute when entering inside the state, and action to execute when exiting from the state, and an action when staying in the state.
- The class
FSMState
extends the abstract FSM node class. - Three attributes are added to store the three actions for the state.
- The getter functions are added for each of the three attributes.
- The setter functions are added for each of the three attributes. Note that the attributes are assumed to be properties of
FSMState
. If the setter functions are firing property-change events when the value of an attribute is changing. - To properly save and load a
FSMState
class from an external file, we must override the functionsgetProperties
andsetProperties
. The values of the three attributes must be replied and set by these functions, respectively. Note that the functionpropGet
is an utility function provided by the super class. It permits to retreive the properties and cast it to the proper type in one call. Do not forget to invoke the super implementations.
1.3.Transition Definition
The FSM transition is defined in the class FSMTransition
. A transition has a name, an action to execute when transition is traversed, and a guard (a condition) that must be true to traverse the transition.
- The
FSMTransition
class extends the classStandardEdge
, which is the implementation of an edge. The generic parameters are the types of the graph, node, anchor and transition supported by the implementation (here the FSM classes). - Three attributes are added to store the action and the guard.
- The getter functions are added for each of the two attributes.
- The setter functions are added for each of the two attributes. Note that the attributes are assumed to be properties of
FSMTransition
. If the setter functions are firing property-change events when the value of an attribute is changing. - To properly save and load a
FSMTransition
class from an external file, we must override the functionsgetProperties
andsetProperties
. The values of the three attributes must be replied and set by these functions, respectively. Note that the functionpropGet
is an utility function provided by the super class. It permits to retreive the properties and cast it to the proper type in one call. Do not forget to invoke the super implementations. - To help the developer to connect the
FSMTransition
to twoFSMState
instances, two helpful functions are added:setStartNode
andsetEndNode
. These functions set the start anchor and the end anchor of the edge to the single anchor of given nodes.
1.4. Start-Point Definition
The FSM start point is the point from which the simulation of the FSM must start. It is not a state by itself, but it is an AbstractFSMNode
because it should be link to other FSM nodes with FSM transitions.
- The class
FSMStartPoint
extends the abstract FSM node class.
1.5. End-Point Definition
The FSM end point is the point at which the simulation of the FSM is stopping. It is not a state by itself, but it is an AbstractFSMNode
because it should be link to other FSM nodes with FSM transitions.
- The class
FSMEndPoint
extends the abstract FSM node class.
1.6. Anchor Definition
The anchor is a construct provided by the NetEditor library to help to define how the edges and the nodes are connected. The implementation of the anchor must defined functions that permits to test if a node and an edge could be connected together.
- The class
FSMAnchor
extends theStandardAnchor
, which is providing a standard implementation for anchors. - The function
canConnectAsStartAnchor
must be overridden to specify when anAbstractFSMNode
can be connected to the start of aFSMTransition
. The start of aFSMTransition
cannot be aFSMEndPoint
. If the current anchor is binded to aFSMStartPoint
then the other side of theFSMTransition
must be aFSMState
to accept the connection. In all the other cases, the connection is accepted. - The function
canConnectAsEndAnchor
must be overridden to specify when anAbstractFSMNode
can be connected to the end of aFSMTransition
. The end of aFSMTransition
cannot be aFSMStartPoint
. If the current anchor is binded to aFSMEndPoint
then the other side of theFSMTransition
must be aFSMState
to accept the connection. In all the other cases, the connection is accepted.
1.7. Finite-State-Machine Graph Definition
The class that is representing the FSM is FiniteStateMachine
. According to the NetEditor library, the class FiniteStateMachine
is a type of graph and it extends StandardGraph
with the appropriate generic parameters.
2. Definition of the language figures
The maven module related to this step is: org.arakhne.neteditor.fsm:fsm-figures
.
All the language constructs should have a figure to be rendered.
2.1. Definition of figure for FSMEndPoint
The figure class FSMEndPoint
extends the class CircleNodeFigure
which the implementation of a circle provided by the NetEditor library.
- The function
paintNode
must be overridden to draw the end point in a proper way. - The graphical context is not a AWT
Graphics2D
instance, but is is aViewGraphics2D
instance. Why? First,Graphics2D
is not dedicated to vectorial drawing, andViewGraphics2D
is. Second,Graphics2D
is an AWT implementation, andViewGraphics2D
is platform-independent (AWT, Android...). - The
ViewGraphics2D
graphical context distinguishes the outline and the interior of a draw. The functiondraw
is able to draw the outline and the interior at the same time. The functionssetInteriorPainted
andsetOutlineDrawn
permits to enable or disable the drawing of the interior and the outline, respectively. In opposite to the famousGraphics2D
, which has one current color, theViewGraphics2D
distinguishes the color used to draw the outline and the color used to draw the interior. - The function
getCurrentViewBounds
permits to retreive the bounds of the figure. - The functions
beginGroup
andendGroup
permits to group the drawn element into a single draw. Grouping is very useful when the figure should be exported into vectorial formats such as SVG.
2.1. Definition of the figures
The figures for the FSMStartPoint
, FSMState
, FSMTransition
, and FSMAnchor
are coded in a similar way.
3. Definition of the figure factory
The maven module related to this step is: org.arakhne.neteditor.fsm:fsm-figures
.
The NetEditor viewer requires to have a figure factory to create the figures when they are not yet associated to graph elements that may be rendered. The class FSMFigureFactory
is the implementation of a figure factory dedicated to the FSM classes. It extends the class AbstractStandardFigureFactory
, whish provides a standard implementation for factories.
- The function
createFigureFor(UUID, FiniteStateMachine, ModelObject, float, float)
is invoked to create a figure for the given model object (which is always a node) at the specified position. The following implementation creates the corresponding figure for the FSM elements. If the figure to create is for aFSMState
, then the function is also creating the figures for theFSMAnchor
. - The function
createFigureFor(UUID, FiniteStateMachine, ModelObject, float, float, float, float)
is invoked to create a figure for the given model object (which is always an edge) from the specified point to the given point. The following implementation creates the corresponding figure for the FSM transition. - The function
createSubFigureInside(UUID, FiniteStateMachine, Figure, ModelObject)
is invoked to create the subfigure inside the given figure for the specified FSM element (usually anFSMAnchor
).
3. Definition of an FSM Editor
The maven module related to this step is: org.arakhne.neteditor.fsm:fsm-editor
.
The FSM editor is a frame that contains the NetEditor viewer, which is an instance of JFigureViewer
.
The standard way to create the editor is illustrated in the following code.